Creating Custom Validators In Reactive Forms Using Angular 6
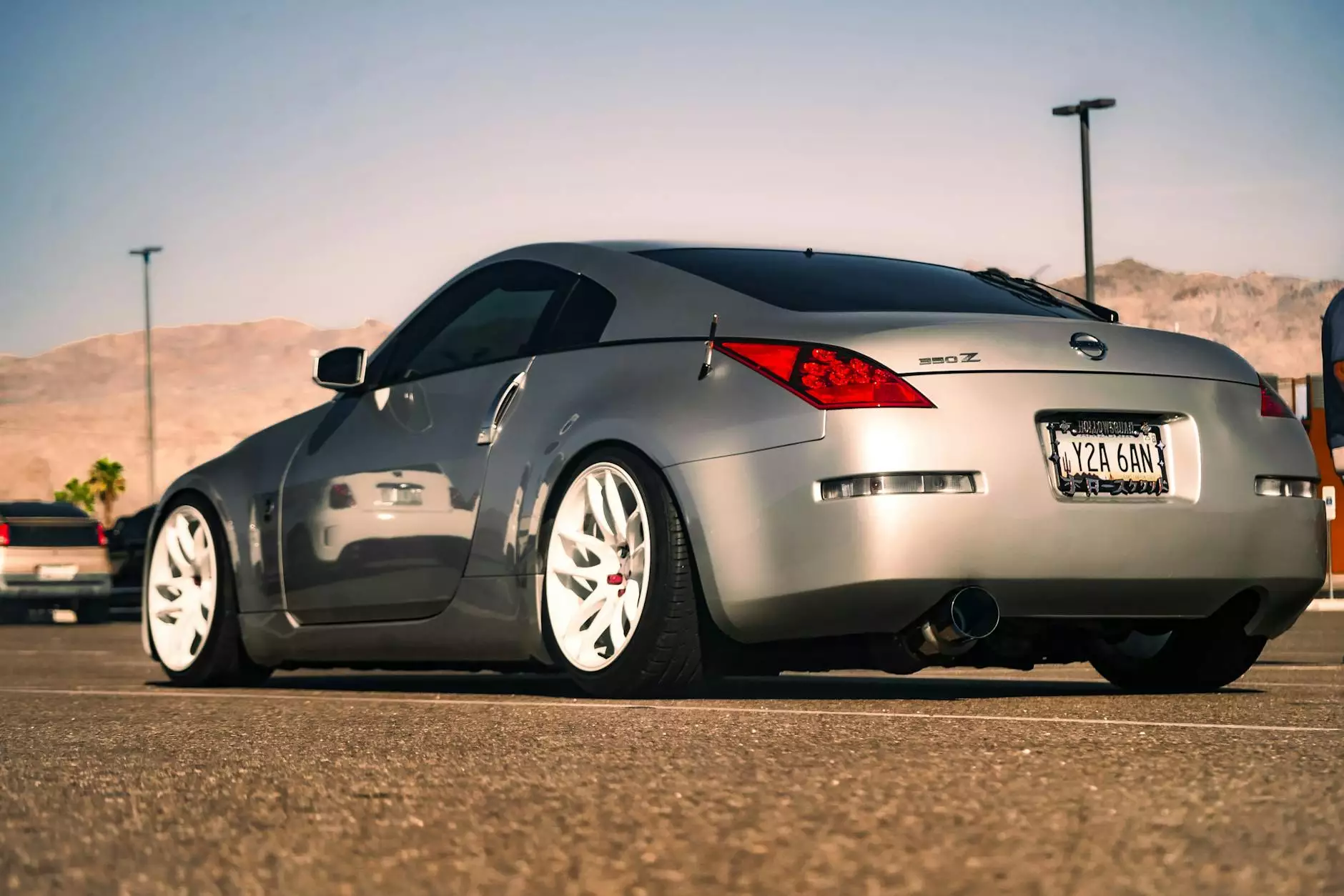
Introduction
Welcome to TECHrx Services, your go-to resource for all things Angular and reactive forms. In this guide, we will walk you through the process of creating custom validators in reactive forms using Angular 6.
What are Reactive Forms?
Reactive forms are a powerful feature in Angular that allow you to build dynamic and interactive user interfaces. They provide a way to define form controls and react to user input, making it easier to implement form validation logic.
Why Custom Validators?
While Angular provides numerous built-in validators, there may be cases where you need to implement custom validation logic based on your specific requirements. Custom validators enable you to add unique validation rules to your reactive forms, ensuring data integrity and user-friendly experiences.
Getting Started
To create custom validators in Angular 6, follow these steps:
- Create a new Angular project using the Angular CLI.
- Import the necessary modules for reactive forms.
- Create a component for your reactive form.
- Define the form controls and validation rules.
- Implement custom validators as per your requirements.
- Apply the custom validators to the respective form controls.
Implementing Custom Validators
Let's dive deeper into the process of implementing custom validators in Angular 6:
Step 1: Import the Required Modules
In your Angular project, make sure to import the following modules:
import { Validators, FormControl } from '@angular/forms';Step 2: Create a Custom Validator Function
Next, define your custom validator function. This function should accept a form control as an argument and return a validation error object if the condition is not met:
function customValidator(control: FormControl): {[key: string]: any} | null { // Custom validation logic here }Step 3: Apply the Custom Validator
To apply the custom validator to a specific form control, use the `Validators` class provided by Angular. Here's an example:
const myForm = new FormGroup({ myControl: new FormControl('', customValidator) });Step 4: Enhancing Custom Validators
You can further enhance your custom validators by adding additional validation rules, such as required fields, minimum and maximum values, or pattern matching. Angular provides a set of pre-built validator functions that you can utilize in combination with your custom validators.
Step 5: Error Handling and User Feedback
When a custom validation fails, you can display an error message to the user by using Angular's built-in error handling capabilities. By associating the error message with the respective form control, users will receive immediate feedback on their input.
Conclusion
Congratulations! You have successfully learned how to create custom validators in reactive forms using Angular 6. Custom validators give you the flexibility to implement unique validation rules for your Angular applications, ensuring data accuracy and user satisfaction.
TECHrx Services - Your Angular Experts
At TECHrx Services, we specialize in providing comprehensive solutions for all your Angular development needs. Whether you need assistance with reactive forms, custom validators, or any other aspect of Angular, our team of experts is ready to help. Contact us today to discuss how we can elevate your Angular projects to new heights.